🔌 Edge function
Invoke a Supabase function from your Bubble workflow.
In this section, we will explore how to use Supabase's serverless edge functions in your Bubble.io application.
Supabase Edge Functions could serve as an alternative for some of your Bubble.io backend workflows. They are simple to write, easy to deploy, and an attractive option with a free tier of up to 500k calls per month.
Deploying an edge function
To get started the first step is to install the Supabase CLI. You can find the instructions here and the Edge function documentation here.
In this section, we will deploy a simple edge function that returns a greeting message.
Step 1 - Create the function hello
Create function
supabase functions new hello
Step 2 - Update the code to handle CORS header
To handle requests from other domains, your edge function needs to include CORS (Cross-Origin Resource Sharing) headers. Without these headers, browsers will block cross-origin requests for security reasons.
Create function
import { serve } from 'https://deno.land/std@0.203.0/http/server.ts'
const corsHeaders = {
'Access-Control-Allow-Origin': '*',
'Access-Control-Allow-Headers':
'authorization, x-client-info, apikey, content-type',
}
serve(async (req) => {
if (req.method === 'OPTIONS') {
return new Response('ok', { headers: corsHeaders })
}
const { name } = await req.json()
const data = {
message: `Hello ${name}!`,
}
return new Response(JSON.stringify(data), {
headers: { ...corsHeaders, 'Content-Type': 'application/json' },
})
})
Step 3 - Deploy the code to Supabase
Deploy function
supabase functions deploy hello --no-verify-jwt
Please note that this code primarily serves as a basic demonstration of how to use Supabase Edge Functions. It may require additional modification to be used in a production environment.
For more detailed information about working with Supabase Edge Functions, you can refer to the official guide available here
Configuring the API Connector
In order to parse the results from the edge function on your Bubble.io app, we will start by setting up the API connector.
- Go to the
API Connector
settings and click onAdd another API
. - Enter a recognizable name for the API, such as
Supabase
. - Add a new API call, such as
Edge Function Hello
- Click on
Manually enter API response
and copy/paste the results format. - Save this response
In the example of the Hello
function, the JSON format is quite simple.
JSON schema
{
"message": ""
}
Setup
Add the element Supabase Edge function
to your page and configure the following field:
- Name
Data Type
- Type
- App Data
- Description
This is the edge function return type.
- Name
Expected response (JSON)
- Type
- JSON
- Description
By default, the plugin attempts to automatically retrieve the expected result based on the type defined in the API connector. To prevent any retrieval failures and ensure that the plugin can properly publish results from Supabase, we recommend you paste your schema into this field. This schema should match the one you have already entered in the API connector.
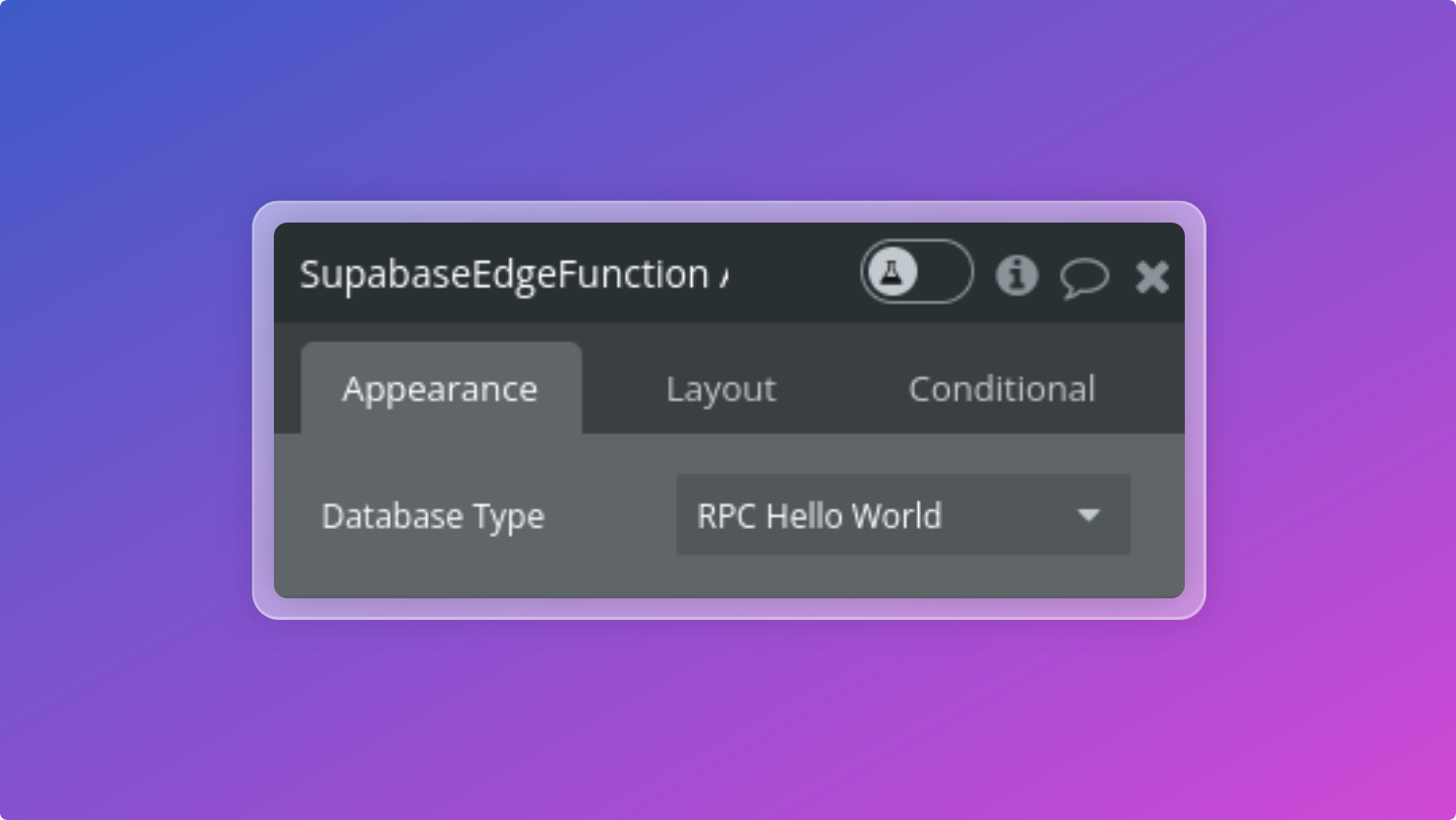
Supabase edge function component
States
The element exposes the following state:
Success
: return "yes" if the function call has been successful.Result
: results returned by the Edge Function.
Events
The element triggers the following event:
Get Result
: triggered when the result has been retrieved
Invoke a function
Invoke a Supabase Edge Function. You can configure the following fields:
- Name
Function Name
- Type
- String
- Description
Name of your deployed Edge Function
- Name
Method
- Type
- Dropdown
- Description
The HTTP verb of the request. Defaults to POST.
- Name
Region
- Type
- Dropdown
- Description
The region to invoke the function in. Defaults to any.
- Name
Headers
- Type
- Array
- Description
Object representing the headers to send with the request. These will be automatically merged with the JSON Headers field.
- Name
JSON Headers
- Type
- JSON
- Description
Object representing the headers in JSON format to send with the request. These will be automatically merged with the Headers field.
- Name
Body
- Type
- JSON
- Description
The body of the request. These will be automatically merged with the JSON Body field.
- Name
JSON Body
- Type
- JSON
- Description
The body of the request in JSON format. These will be automatically merged with the Body field.
Do you need any help? Feel free to reach out