💾 RPC
Invoke database functions from within your Bubble.io app.
In this section, we'll explore the process of invoking database functions provided by Supabase from within your Bubble.io app.
Please ensure that a Supabase Auth component is visible on every page of your app. This is crucial because this component initiates the connection with your Supabase instance.
Setup
Add the element Supabase RPC
to your page.
You can use a separate RPC component for each functions that you wish to call within your Bubble.io app.
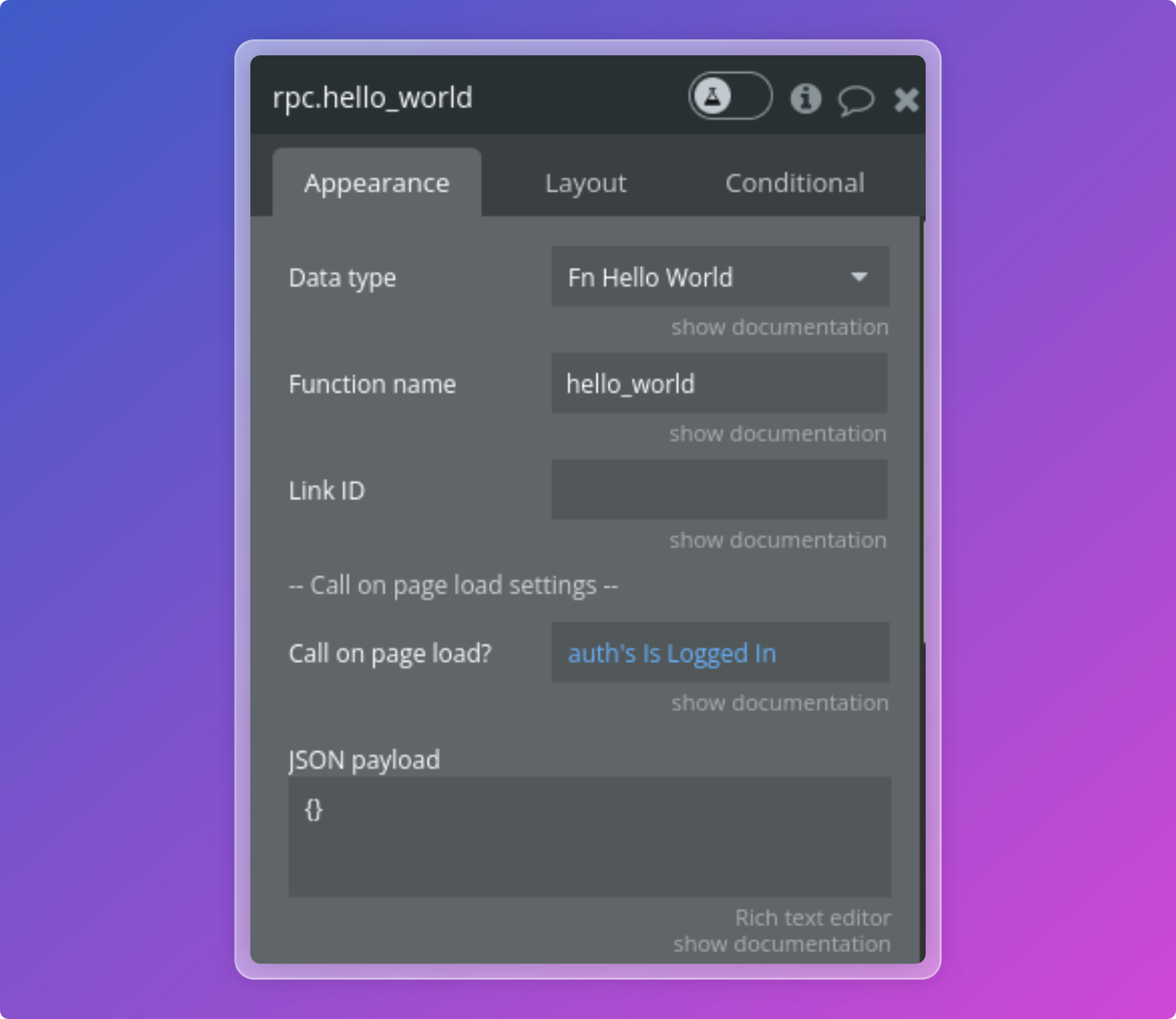
You can configure the following fields:
- Name
DB schema
- Type
- App type
- Description
The Postgres schema which this function belong to.
- Name
Function name
- Type
- text
- Description
Supabase function name.
- Name
Expected type
- Type
- Dropdown
- Description
Defines the type of data returned by the RPC function. Can be: JSON objects, Numbers, Texts, Booleans or Dates. When the RPC function returns a list of objects, select "JSON objects." In this case, you must also define the "Data Type" and "Expected response (JSON object)" fields below.
- Name
Datatype
- Type
- App Data
- Description
Only when the "Expected type" is set to "JSON objects". You must first initialize the API Connector in order to select the correct type.
Please refer to this section for detailed instructions.
- Name
Expected response (JSON object)
- Type
- Text
- Description
By default, the plugin attempts to automatically retrieve the expected result based on the type defined in the API connector. To prevent any retrieval failures and ensure that the plugin can properly publish results from Supabase, we recommend you paste your schema into this field. This schema should match the one you have already entered in the API connector.
Call settings
- Name
Call on page load?
- Type
- Boolean
- Description
Automatically call this function when the page loads. You can still call this function at anytime using the "RPC" action in your workflow.
- Name
JSON payload
- Type
- text
- Description
The arguments to pass to the function call in JSON format.
- Name
Filters
- Description
Please check this section for more details.
- Name
Nulls first?
- Type
- Boolean
- Description
If true, null values are treated as the lowest possible values when sorting in ascending order and as the highest possible values when sorting in descending order. This applies to all columns where ordering is enabled. The default is true.
- Name
Order By
- Type
- text
- Description
The column to order by. Leave empty to not order results.
- Name
Ascending order?
- Type
- Boolean
- Description
When set to true, the query will use an ascending order for this column.
- Name
Limit Count
- Type
- Number
- Description
The maximum number of rows to return. Setting this to 0 does not apply any limit.
- Name
Limit By Range
- Type
- Boolean
- Description
When set to true, the query will limit results to a specific range defined by 'Limit From' and 'Limit To'.
- Name
Limit From
- Type
- Number
- Description
The starting index from which to limit the result. This index is 0-based, meaning an index of 0 refers to the first record.
- Name
Limit To
- Type
- Number
- Description
The last index to which to limit the result. This is inclusive, so the record at this index will be included in the result.
- Name
Count
- Type
- Dropdown
- Description
Count algorithm to use to count rows returned by the function. Only applicable for "set-returning functions".
- Name
Head
- Type
- Boolean
- Description
When set to
true
, thedata
will not be returned. Useful if you only need the count.
- Name
Convert to null
- Type
- Text
- Description
A list of fields that should have empty values (e.g., "") converted to null before inserting into your table. This is useful for columns that expect non-string types, like dates, where an empty string would cause an error.
States
The element exposes the following states:
Objects
: Contain the objects retrieved from your Supabase instance.Object
: in case the results contain only one object, this field will contain that specific object retrieved from your function call.Count
: number of rows when using the options countValue (number)
: the value returned by the function call when the expected type is set to "Number".Value (list of numbers)
: the values returned by the function call when the expected type is set to "Number".Value (text)
: the value returned by the function call when the expected type is set to "Text".Value (list of texts)
: the values returned by the function call when the expected type is set to "Text".Value (boolean)
: the value returned by the function call when the expected type is set to "Boolean".Value (list of booleans)
: the values returned by the function call when the expected type is set to "Boolean".Value (date)
: the value returned by the function call when the expected type is set to "Date".Value (list of dates)
: the values returned by the function call when the expected type is set to "Date".Status Code
: the status code will be '200' in case of success, and a different code in case of an error.Status Message
: the status message will be 'success' in cases of success, and it will contain error details in cases of an error.Is success?
: a boolean that indicates if the function call was successful.
Events
The element triggers the following event:
Call success
: received a successfull response from Supabase.Call error
: received an error from Supabase
RPC
Perform a function call (RPC) wich allows you to execute logic in your database.
You can configure the following field:
- Name
Payload
- Type
- Array
- Description
The arguments to pass to the function call. These will be automatically merged with the JSON payload field.
- Name
JSON Payload
- Type
- JSON
- Description
The arguments to pass to the function call in JSON format. These will be automatically merged with the payload field.
- Name
Filters
- Description
Please check this section for more details.
- Name
Nulls first?
- Type
- Boolean
- Description
If true, null values are treated as the lowest possible values when sorting in ascending order and as the highest possible values when sorting in descending order. This applies to all columns where ordering is enabled. The default is true.
- Name
Order By
- Type
- text
- Description
The column to order by. Leave empty to not order results.
- Name
Ascending order?
- Type
- Boolean
- Description
When set to true, the query will use an ascending order for this column.
- Name
Limit Count
- Type
- Number
- Description
The maximum number of rows to return. Setting this to 0 does not apply any limit.
- Name
Limit By Range
- Type
- Boolean
- Description
When set to true, the query will limit results to a specific range defined by 'Limit From' and 'Limit To'.
- Name
Limit From
- Type
- Number
- Description
The starting index from which to limit the result. This index is 0-based, meaning an index of 0 refers to the first record.
- Name
Limit To
- Type
- Number
- Description
The last index to which to limit the result. This is inclusive, so the record at this index will be included in the result.
- Name
Count
- Type
- Dropdown
- Description
Count algorithm to use to count rows returned by the function.
exact
: Exact but slow count algorithm. Performs aCOUNT(*)
under the hood.planned
: Approximated but fast count algorithm. Uses the Postgres statistics under the hood.estimated
: Uses exact count for low numbers and planned count for high numbers.
- Name
Head
- Type
- Boolean
- Description
When set to
true
,data
will not be returned. Useful if you only need the count.
- Name
Convert to null
- Type
- Text
- Description
A list of fields that should have empty values (e.g., "") converted to null before inserting into your table. This is useful for columns that expect non-string types, like dates, where an empty string would cause an error.
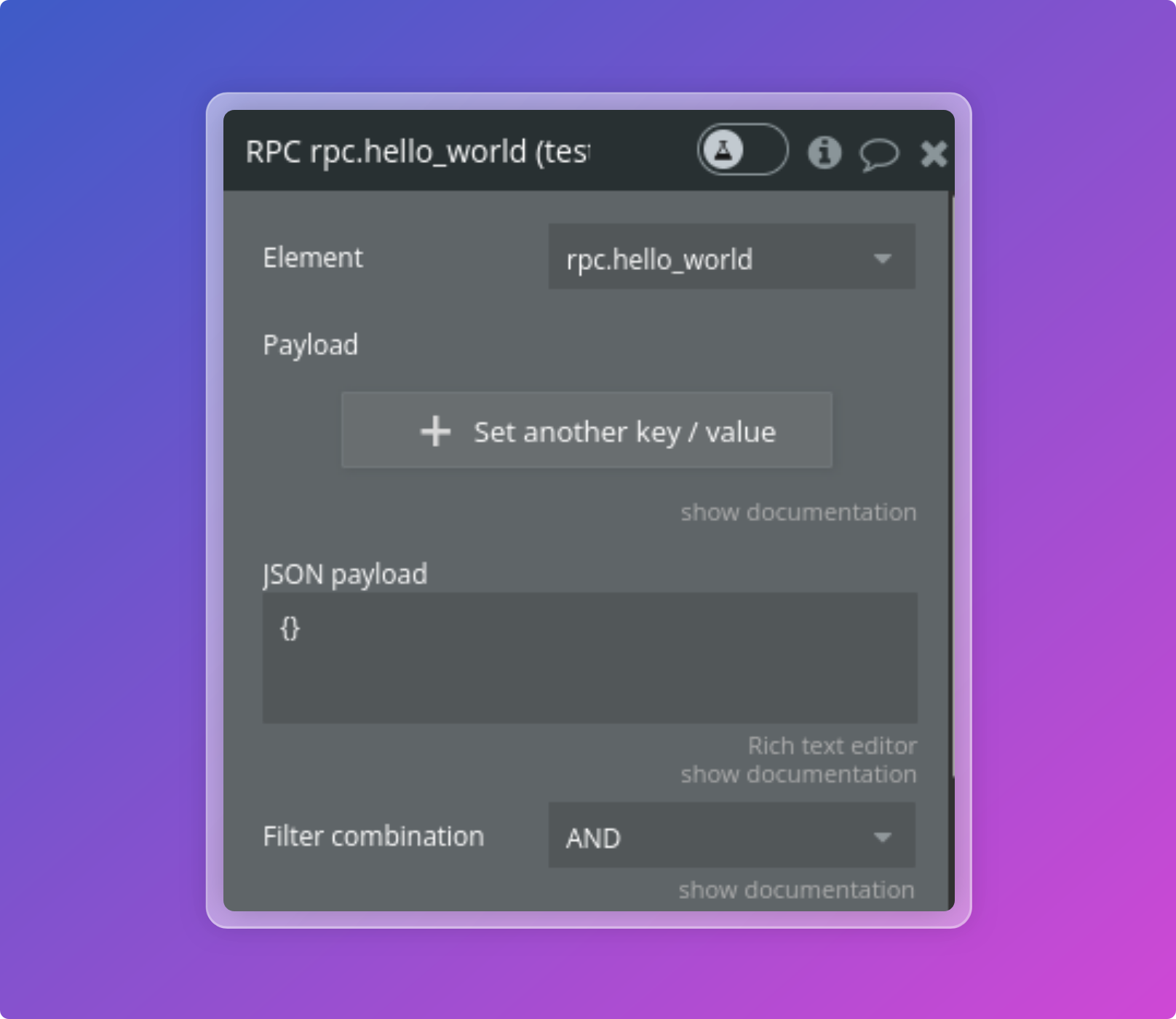
Perform a function call (RPC)
RPC example
We'll create the function hello_world_with_name
that takes a single text argument name,
and returns a JSON object containing a greeting message concatenated with the
provided name.
1 - Create the RPC function on Supabase
Function
CREATE OR REPLACE FUNCTION hello_world_with_name(name text)
RETURNS json AS $$
BEGIN
RETURN json_build_object('message', 'Hello world ' || name);
END;
$$ LANGUAGE plpgsql;
2 - Paste the schema to the Bubble.io API Connector
Our function will returns the following JSON schema:
{
"message": ""
}
All we need to do is to initialize the API connector in order to use the format in our RPC component:
3 - Initializing the RPC component
You can now add an RPC component to your page:
You can then retrieve the result of the call from the Object
state in your RPC component.
Do you need any help? Feel free to reach out